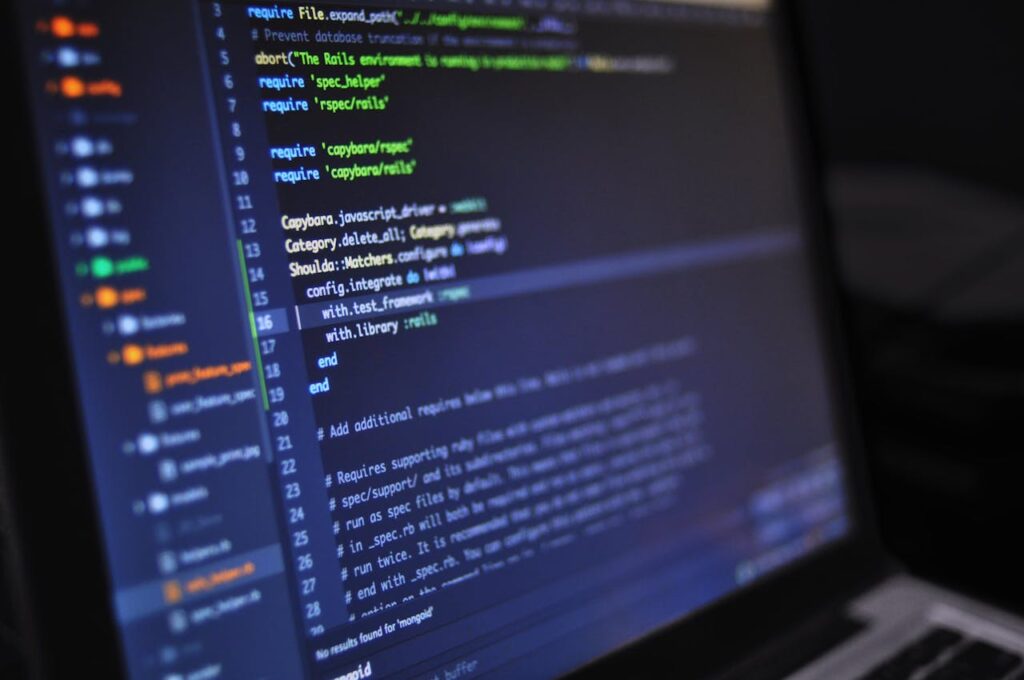
JavaScript is a language heavily driven by asynchronous operations, meaning tasks like network requests, file handling, and database interactions don’t halt the execution of other code. Handling these operations requires mechanisms that allow you to run tasks in the background while the rest of your program continues to execute. Two of the most powerful tools for managing asynchronous code in JavaScript are Promises and Async/Await.
In this comprehensive guide, we’ll dive deep into understanding JavaScript Promises, the core of asynchronous handling, and how async/await
simplifies their usage. By the end, you’ll have a firm grasp of these concepts, enabling you to write more readable and efficient code.
Table of Contents
- What is Asynchronous Programming?
- Callbacks: The Old Way
- Introduction to JavaScript Promises
- Creating a Promise
- The
then()
andcatch()
Methods - Promise States
- Chaining Promises
- Common Pitfalls with Promises
Async
andAwait
Explained- Why
Async/Await
? - Writing
Async/Await
Code
- Why
- Handling Errors with
Async/Await
- Working with Multiple Promises
Promise.all()
Promise.race()
- Best Practices for Using Promises and
Async/Await
- Conclusion
1. What is Asynchronous Programming?
Asynchronous programming allows tasks to run in the background without blocking the main thread. For instance, in a synchronous system, if you request data from an API, the entire script halts until the data is retrieved. However, in an asynchronous system, the script can continue running while waiting for the data, making the code non-blocking.
JavaScript handles asynchronous operations primarily through callbacks, Promises, and async/await
.
2. Callbacks: The Old Way
Before Promises, asynchronous tasks were handled using callbacks. A callback is a function passed as an argument to another function, executed when the asynchronous task is completed.
javascriptCopy codefunction fetchData(callback) {
setTimeout(() => {
callback("Data received");
}, 2000);
}
fetchData((data) => {
console.log(data); // "Data received" after 2 seconds
});
While callbacks work, they quickly become problematic when you need to perform multiple asynchronous operations in sequence, leading to callback hell, a situation where code becomes nested and difficult to read.
3. Introduction to JavaScript Promises
Promises are a modern solution to handling asynchronous tasks. A Promise represents a value that may be available now, in the future, or never. In other words, it acts as a placeholder for the result of an asynchronous operation.
Creating a Promise
A Promise is created using the Promise
constructor, which takes a function with two arguments: resolve
and reject
. resolve
is called when the asynchronous task is successful, while reject
is called when there is an error.
javascriptCopy codelet promise = new Promise((resolve, reject) => {
let success = true;
if (success) {
resolve("Task completed successfully");
} else {
reject("Task failed");
}
});
The then()
and catch()
Methods
To handle the result of a Promise, we use the .then()
method. This method takes a function as an argument, which is executed when the Promise is resolved. If an error occurs, the .catch()
method is used to handle the rejection.
javascriptCopy codepromise.then((message) => {
console.log(message); // "Task completed successfully"
}).catch((error) => {
console.log(error);
});
Promise States
A Promise can be in one of three states:
- Pending: The asynchronous operation is still in progress.
- Fulfilled: The operation was successful, and
resolve()
was called. - Rejected: The operation failed, and
reject()
was called.
Chaining Promises
One of the powerful features of Promises is their ability to chain .then()
calls. This is useful when you need to perform a series of asynchronous tasks sequentially.
javascriptCopy codelet promise = new Promise((resolve) => {
resolve(10);
});
promise
.then((num) => {
console.log(num); // 10
return num * 2;
})
.then((num) => {
console.log(num); // 20
return num * 2;
})
.then((num) => {
console.log(num); // 40
});
This chaining mechanism prevents deeply nested callbacks and keeps the code clean and readable.
4. Common Pitfalls with Promises
While Promises simplify asynchronous code, they come with challenges:
- Error handling: A failure at any point in a Promise chain can break the entire chain if not properly handled.
- Uncaught rejections: Failing to catch errors can result in uncaught Promise rejections.
- Readability: Despite being cleaner than callbacks, multiple chained
.then()
can still get messy with complex logic.
5. Async
and Await
Explained
To overcome the challenges of Promises, ES2017 introduced async
and await
, which make asynchronous code look synchronous and improve readability.
Why Async/Await
?
With async/await
, you can write asynchronous code in a synchronous manner. This improves clarity, especially when performing multiple asynchronous operations in sequence.
Writing Async/Await
Code
An async
function is a function that returns a Promise. Inside an async
function, the await
keyword is used to pause the execution of the function until the Promise is resolved.
javascriptCopy codeasync function fetchData() {
let promise = new Promise((resolve) => {
setTimeout(() => resolve("Data received"), 2000);
});
let result = await promise; // Pauses until the promise resolves
console.log(result); // "Data received"
}
fetchData();
6. Handling Errors with Async/Await
Error handling in async/await
is simplified using try/catch
blocks. Instead of chaining .catch()
, you wrap your await
statements in a try/catch
block to manage errors.
javascriptCopy codeasync function fetchData() {
try {
let promise = new Promise((resolve, reject) => {
reject("Error occurred");
});
let result = await promise;
console.log(result);
} catch (error) {
console.log(error); // "Error occurred"
}
}
fetchData();
7. Working with Multiple Promises
Sometimes, you need to handle multiple Promises simultaneously. This is where functions like Promise.all()
and Promise.race()
come in.
Promise.all()
Promise.all()
is used to execute multiple Promises in parallel. It returns a single Promise that resolves when all input Promises are resolved, or rejects if any of them fails.
javascriptCopy codelet promise1 = Promise.resolve(1);
let promise2 = Promise.resolve(2);
let promise3 = Promise.resolve(3);
Promise.all([promise1, promise2, promise3]).then((values) => {
console.log(values); // [1, 2, 3]
});
Promise.race()
Promise.race()
returns a Promise that resolves or rejects as soon as one of the input Promises is settled.
javascriptCopy codelet promise1 = new Promise((resolve) => setTimeout(resolve, 500, 'one'));
let promise2 = new Promise((resolve) => setTimeout(resolve, 100, 'two'));
Promise.race([promise1, promise2]).then((value) => {
console.log(value); // "two" (the fastest promise)
});
8. Best Practices for Using Promises and Async/Await
Here are a few tips to keep in mind:
- Always handle errors: Use
.catch()
with Promises ortry/catch
withasync/await
. - Use
Promise.all()
wisely: It’s ideal when Promises are independent and don’t rely on one another. - Avoid unnecessary
await
: Don’tawait
a value that doesn’t need to be awaited. - Use
async/await
for cleaner code: It provides a cleaner, more readable structure than chaining multiple.then()
.
9. Conclusion
Both Promises and async/await
provide elegant solutions for managing asynchronous code in JavaScript. Promises offer a robust foundation, while async/await
builds on top of that foundation to offer a more readable and maintainable syntax. Whether you’re handling API requests, performing file operations, or running background tasks, understanding these concepts will make your code more efficient, easier to debug, and more scalable.
By mastering Promises and async/await
, you can write asynchronous JavaScript with confidence and build more responsive applications.